An in memory database is created when an application starts up and destroyed when the application is stopped.
That means there is no persistence of data and every time you restart or stop you application your saved data will be lost.
H2
H2 is one of the popular in memory databases. Spring Boot has very good integration for H2.
H2 is a relational database management system written in Java. It can be embedded in Java applications or run in the client-server mode.
H2 also provides a web console to maintain the database.
Setting up a Spring Boot Project with H2
Spring Initializer http://start.spring.io/ is great tool to bootstrap your Spring Boot projects.
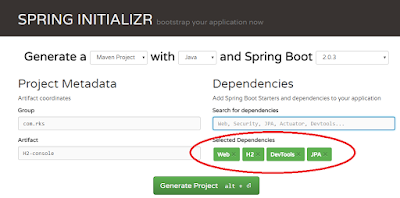
Launch Spring Initializer and choose the following
1. Choose com.rks as Group
2. Choose H2-console as Artifact
3. Choose following dependencies
- Web
- JPA
- H2
- DevTools
Click Generate Project.
Import the project into Eclipse.
File -> Import -> Existing Maven Project.
Create a simple Employee Entity with a primary key id.
package com.rks.h2console; import javax.persistence.*; @Entity @Table(name = "employee") public class Employee { @Id @Column @GeneratedValue(strategy = GenerationType.IDENTITY) private int id; @Column private String firstName; @Column private String lastName; @Column private String email; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
Edit properties file placed in
/src/main/resources/application.properties
# Enabling H2 Console spring.h2.console.enabled=true # Show all queries spring.jpa.show-sql=true #DB configurations spring.datasource.url=jdbc:h2:mem:rksdb spring.datasource.driverClassName=org.h2.Driver spring.datasource.username=test spring.datasource.password=test spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
Run your application from file EmployeePortalApplication.
Right click -> RunAs ->JavaApplication
package com.rks.h2console; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class EmployeePortalApplication { public static void main(String[] args) { SpringApplication.run(EmployeePortalApplication.class, args); } }
When you reload the application, you can launch up H2 Console at http://localhost:8080/h2-console.
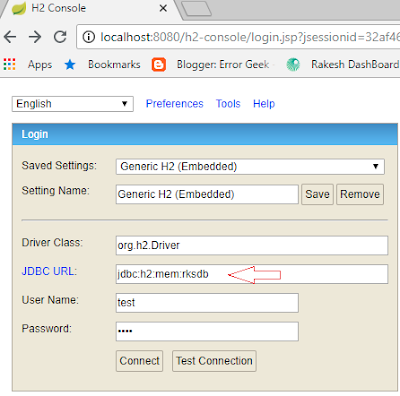
As soon as Spring Boot sees H2 in the class path, it auto configures a data source It knows that you are using an in memory database H2 and it uses the default url if you don’t provide one.
All tables will automatically get created by hibernate corresponding to the beans you have created but if you want to create table using sql scripts
You can also populate some data into student table by adding a file called data.sql
/src/main/resources/data.sql
create table student ( id integer not null, name varchar(255) not null, email varchar(255) not null, primary key(id) ); insert into student values(101,'Rakesh', 'rks@r.com'); insert into student values(102,'Moni', 'moni@m.com'); commit;
Source code
https://github.com/rsinghania/H2-Console-Db.git
Comments
Post a Comment